Start Google Maps using intent of ACTION_VIEW, with zoom level.
To start Google Maps using intent of ACTION_VIEW with zoom level, you can use with the Uri:
geo:Latitude,Longitude?z=<zoom level>
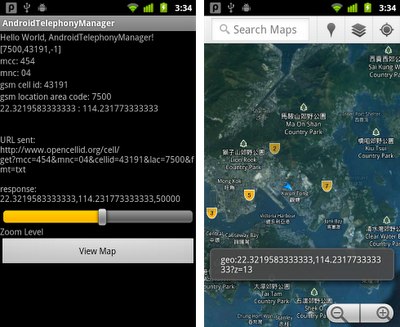
Modify the main.xml in last post "Start Google Maps using intent of ACTION_VIEW", include a SeekBar for user to set zoom level.
Modify btnViewMap.setOnClickListener() to start activity with Uri with zoom level
geo:Latitude,Longitude?z=<zoom level>
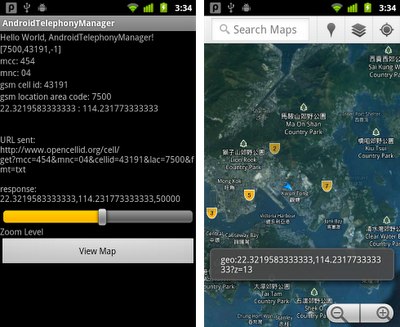
Modify the main.xml in last post "Start Google Maps using intent of ACTION_VIEW", include a SeekBar for user to set zoom level.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
| <? xml version = "1.0" encoding = "utf-8" ?> android:orientation = "vertical" android:layout_width = "fill_parent" android:layout_height = "fill_parent" > < TextView android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:text = "@string/hello" /> < TextView android:id = "@+id/gsmcelllocation" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/mcc" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/mnc" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/cid" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/lac" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/geo" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/remark" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < SeekBar android:id = "@+id/zoom" android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:paddingTop = "5dp" android:paddingLeft = "5dp" android:paddingRight = "5dp" android:max = "21" /> < TextView android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:text = "Zoom Level" /> < Button android:id = "@+id/viewmap" android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:text = "View Map" /> </ LinearLayout > |
Modify btnViewMap.setOnClickListener() to start activity with Uri with zoom level
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
| package com.AndroidTelephonyManager; import org.apache.http.HttpResponse; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.DefaultHttpClient; import org.apache.http.util.EntityUtils; import android.app.Activity; import android.content.Context; import android.content.Intent; import android.net.Uri; import android.os.Bundle; import android.telephony.TelephonyManager; import android.telephony.gsm.GsmCellLocation; import android.view.View; import android.widget.Button; import android.widget.SeekBar; import android.widget.TextView; import android.widget.Toast; public class AndroidTelephonyManager extends Activity { public class OpenCellID { String mcc; //Mobile Country Code String mnc; //mobile network code String cellid; //Cell ID String lac; //Location Area Code Boolean error; String strURLSent; String GetOpenCellID_fullresult; String latitude; String longitude; public Boolean isError(){ return error; } public void setMcc(String value){ mcc = value; } public void setMnc(String value){ mnc = value; } public void setCallID( int value){ cellid = String.valueOf(value); } public void setCallLac( int value){ lac = String.valueOf(value); } public String getLocation(){ return (latitude + " : " + longitude); } public String getLatitude(){ return latitude; } public String getLongitude(){ return longitude; } public void groupURLSent(){ strURLSent = + "&mnc=" + mnc + "&cellid=" + cellid + "&lac=" + lac + "&fmt=txt" ; } public String getstrURLSent(){ return strURLSent; } public String getGetOpenCellID_fullresult(){ return GetOpenCellID_fullresult; } public void GetOpenCellID() throws Exception { groupURLSent(); HttpClient client = new DefaultHttpClient(); HttpGet request = new HttpGet(strURLSent); HttpResponse response = client.execute(request); GetOpenCellID_fullresult = EntityUtils.toString(response.getEntity()); spliteResult(); } private void spliteResult(){ if (GetOpenCellID_fullresult.equalsIgnoreCase( "err" )){ error = true ; } else { error = false ; String[] tResult = GetOpenCellID_fullresult.split( "," ); latitude = tResult[ 0 ]; longitude = tResult[ 1 ]; } } } int myLatitude, myLongitude; OpenCellID openCellID; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.main); TextView textGsmCellLocation = (TextView)findViewById(R.id.gsmcelllocation); TextView textMCC = (TextView)findViewById(R.id.mcc); TextView textMNC = (TextView)findViewById(R.id.mnc); TextView textCID = (TextView)findViewById(R.id.cid); TextView textLAC = (TextView)findViewById(R.id.lac); TextView textGeo = (TextView)findViewById(R.id.geo); TextView textRemark = (TextView)findViewById(R.id.remark); final SeekBar zoomBar = (SeekBar)findViewById(R.id.zoom); Button btnViewMap = (Button)findViewById(R.id.viewmap); btnViewMap.setOnClickListener( new Button.OnClickListener(){ @Override public void onClick(View arg0) { // TODO Auto-generated method stub int zoom = zoomBar.getProgress()+ 2 ; //convert to 2..23 //set 1 will be Force Closed! I don't know why. //geo:Latitude,Longitude?z=<zoom level> String stringLoc = "geo:" + openCellID.getLatitude() + "," + openCellID.getLongitude() + "?z=" + String.valueOf(zoom); Toast.makeText(AndroidTelephonyManager. this , stringLoc, Toast.LENGTH_LONG).show(); Intent intent = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse(stringLoc)); startActivity(intent); }}); //retrieve a reference to an instance of TelephonyManager TelephonyManager telephonyManager = (TelephonyManager)getSystemService(Context.TELEPHONY_SERVICE); GsmCellLocation cellLocation = (GsmCellLocation)telephonyManager.getCellLocation(); String networkOperator = telephonyManager.getNetworkOperator(); String mcc = networkOperator.substring( 0 , 3 ); String mnc = networkOperator.substring( 3 ); textMCC.setText( "mcc: " + mcc); textMNC.setText( "mnc: " + mnc); int cid = cellLocation.getCid(); int lac = cellLocation.getLac(); textGsmCellLocation.setText(cellLocation.toString()); textCID.setText( "gsm cell id: " + String.valueOf(cid)); textLAC.setText( "gsm location area code: " + String.valueOf(lac)); openCellID = new OpenCellID(); openCellID.setMcc(mcc); openCellID.setMnc(mnc); openCellID.setCallID(cid); openCellID.setCallLac(lac); try { openCellID.GetOpenCellID(); if (!openCellID.isError()){ textGeo.setText(openCellID.getLocation()); textRemark.setText( "\n\n" + "URL sent: \n" + openCellID.getstrURLSent() + "\n\n" + "response: \n" + openCellID.GetOpenCellID_fullresult); } else { textGeo.setText( "Error" ); } } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); textGeo.setText( "Exception: " + e.toString()); } } }
akm www.cdacians.com |
No comments:
Post a Comment