Get location of Cell ID, from opencellid.org using HttpGet().
A class OpenCellID was created to handle the http communication with opencellid.org. To simplify the job, request with "fmt=txt" is sent, such that we can simply splite the result to retrieve our latitude, longitude.
To know more about the project OpenCellID, refer to last post.
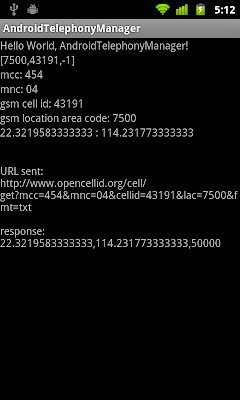
We need the following permission in this example:
To know more about the project OpenCellID, refer to last post.
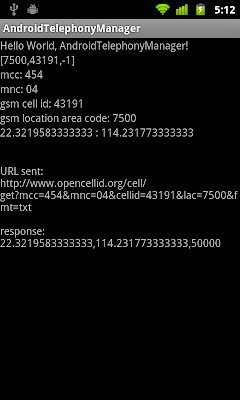
We need the following permission in this example:
- android.permission.ACCESS_COARSE_LOCATION
- android.permission.ACCESS_FINE_LOCATION
- android.permission.READ_PHONE_STATE
- android.permission.INTERNET
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
| package com.AndroidTelephonyManager; import org.apache.http.HttpResponse; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.DefaultHttpClient; import org.apache.http.util.EntityUtils; import android.app.Activity; import android.content.Context; import android.os.Bundle; import android.telephony.TelephonyManager; import android.telephony.gsm.GsmCellLocation; import android.widget.TextView; public class AndroidTelephonyManager extends Activity { public class OpenCellID { String mcc; //Mobile Country Code String mnc; //mobile network code String cellid; //Cell ID String lac; //Location Area Code Boolean error; String strURLSent; String GetOpenCellID_fullresult; String latitude; String longitude; public Boolean isError(){ return error; } public void setMcc(String value){ mcc = value; } public void setMnc(String value){ mnc = value; } public void setCallID( int value){ cellid = String.valueOf(value); } public void setCallLac( int value){ lac = String.valueOf(value); } public String getLocation(){ return (latitude + " : " + longitude); } public void groupURLSent(){ strURLSent = + "&mnc=" + mnc + "&cellid=" + cellid + "&lac=" + lac + "&fmt=txt" ; } public String getstrURLSent(){ return strURLSent; } public String getGetOpenCellID_fullresult(){ return GetOpenCellID_fullresult; } public void GetOpenCellID() throws Exception { groupURLSent(); HttpClient client = new DefaultHttpClient(); HttpGet request = new HttpGet(strURLSent); HttpResponse response = client.execute(request); GetOpenCellID_fullresult = EntityUtils.toString(response.getEntity()); spliteResult(); } private void spliteResult(){ if (GetOpenCellID_fullresult.equalsIgnoreCase( "err" )){ error = true ; } else { error = false ; String[] tResult = GetOpenCellID_fullresult.split( "," ); latitude = tResult[ 0 ]; longitude = tResult[ 1 ]; } } } int myLatitude, myLongitude; OpenCellID openCellID; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.main); TextView textGsmCellLocation = (TextView)findViewById(R.id.gsmcelllocation); TextView textMCC = (TextView)findViewById(R.id.mcc); TextView textMNC = (TextView)findViewById(R.id.mnc); TextView textCID = (TextView)findViewById(R.id.cid); TextView textLAC = (TextView)findViewById(R.id.lac); TextView textGeo = (TextView)findViewById(R.id.geo); TextView textRemark = (TextView)findViewById(R.id.remark); //retrieve a reference to an instance of TelephonyManager TelephonyManager telephonyManager = (TelephonyManager)getSystemService(Context.TELEPHONY_SERVICE); GsmCellLocation cellLocation = (GsmCellLocation)telephonyManager.getCellLocation(); String networkOperator = telephonyManager.getNetworkOperator(); String mcc = networkOperator.substring( 0 , 3 ); String mnc = networkOperator.substring( 3 ); textMCC.setText( "mcc: " + mcc); textMNC.setText( "mnc: " + mnc); int cid = cellLocation.getCid(); int lac = cellLocation.getLac(); textGsmCellLocation.setText(cellLocation.toString()); textCID.setText( "gsm cell id: " + String.valueOf(cid)); textLAC.setText( "gsm location area code: " + String.valueOf(lac)); openCellID = new OpenCellID(); openCellID.setMcc(mcc); openCellID.setMnc(mnc); openCellID.setCallID(cid); openCellID.setCallLac(lac); try { openCellID.GetOpenCellID(); if (!openCellID.isError()){ textGeo.setText(openCellID.getLocation()); textRemark.setText( "\n\n" + "URL sent: \n" + openCellID.getstrURLSent() + "\n\n" + "response: \n" + openCellID.GetOpenCellID_fullresult); } else { textGeo.setText( "Error" ); } } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); textGeo.setText( "Exception: " + e.toString()); } } } |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
| <? xml version = "1.0" encoding = "utf-8" ?> android:orientation = "vertical" android:layout_width = "fill_parent" android:layout_height = "fill_parent" > < TextView android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:text = "@string/hello" /> < TextView android:id = "@+id/gsmcelllocation" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/mcc" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/mnc" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/cid" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/lac" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/geo" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> < TextView android:id = "@+id/remark" android:layout_width = "fill_parent" android:layout_height = "wrap_content" /> </ LinearLayout >
akm www.cdacians.com |
No comments:
Post a Comment