Through this application we can populate a google map with the info window, zoom controls and marker features. The image of the running application is shown below
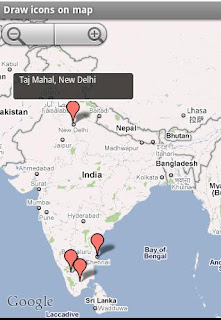
First of all, we can run the application with the google api sdk only, we can use from API level 3, but the Google api sdk should be used to run the application.
LocationViewers.java
In this LocationViewers.java only we are using the MapView, then this file will be called in the map.xml
map.xml
In the above mentioned xml file we had used the ZoomControls also, this will be given the zoom listener on the java file
DrawIcons.java
While pressing the zoom controls plus or minus, this listener will be called and the zoom functionality will be occurs. Then we are going to see two filesMapLocation.java and MapLocationOverlay.java, where in the MapLocationOverlay file we will be drawing the marker and the info window for each marker, you can get the code from the zip file below.
The main thing to run the application is adding the internet permission and google library in the AndroidManifest.xml file
AndroidManifest.xml
First of all, we can run the application with the google api sdk only, we can use from API level 3, but the Google api sdk should be used to run the application.
Custom Linear layout is used on this application
LocationViewers.java
package com.icons.draw.view; import java.util.ArrayList; import java.util.List; import android.content.Context; import android.util.AttributeSet; import android.view.View; import android.widget.LinearLayout; import android.widget.ZoomControls; import com.google.android.maps.MapController; import com.google.android.maps.MapView; import com.icons.draw.R; public class LocationViewers extends LinearLayout { private MapLocationOverlay overlay; // Known lat/long coordinates that we'll be using. private ListmapLocations; public static MapView mapView; public LocationViewers(Context context, AttributeSet attrs) { super(context, attrs); init(); } public LocationViewers(Context context) { super(context); init(); } public void init() { setOrientation(VERTICAL); setLayoutParams(new LinearLayout.LayoutParams(android.view.ViewGroup.LayoutParams.FILL_PARENT,android.view.ViewGroup.LayoutParams.FILL_PARENT)); String api = getResources().getString(R.string.map_api_key); mapView = new MapView(getContext(),api); mapView.setEnabled(true); mapView.setClickable(true); addView(mapView); overlay = new MapLocationOverlay(this); mapView.getOverlays().add(overlay); mapView.getController().setZoom(5); mapView.getController().setCenter(getMapLocations().get(0).getPoint()); } public List getMapLocations() { if (mapLocations == null) { mapLocations = new ArrayList (); mapLocations.add(new MapLocation("Avinashi road, Coimbatore",11.0138,76.9871)); mapLocations.add(new MapLocation("Marina Beach, Chennai",13.0548,80.2830)); mapLocations.add(new MapLocation("Taj Mahal, New Delhi",28.6353,77.2250)); mapLocations.add(new MapLocation("Meenakshi Temple, Madurai",9.9195,78.1208)); } return mapLocations; } public MapView getMapView() { return mapView; } }
In this LocationViewers.java only we are using the MapView, then this file will be called in the map.xml
map.xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/home_container" android:layout_width="fill_parent"
android:layout_height="fill_parent">
<com.icons.draw.view.LocationViewers
android:id="@+id/map_location_viewer" android:layout_width="fill_parent"
android:layout_height="fill_parent" >
</com.icons.draw.view.LocationViewers>
<ZoomControls android:id="@+id/zoomcontrols"
android:gravity="bottom"
android:layout_width="wrap_content" android:layout_height="wrap_content" />
</FrameLayout>
In the above mentioned xml file we had used the ZoomControls also, this will be given the zoom listener on the java file
DrawIcons.java
package com.icons.draw; import com.google.android.maps.MapActivity; import android.os.Bundle; import android.view.View; import android.widget.LinearLayout; import android.widget.ZoomControls; import com.icons.draw.view.LocationViewers; public class DrawIcons extends MapActivity { @Override public void onCreate(Bundle icicle) { super.onCreate(icicle); setContentView(R.layout.map); ZoomControls zoomControls = (ZoomControls) findViewById(R.id.zoomcontrols); zoomControls.setOnZoomInClickListener(new View.OnClickListener() { @Override public void onClick(View v) { LocationViewers.mapView.getController().zoomIn(); } }); zoomControls.setOnZoomOutClickListener(new View.OnClickListener() { @Override public void onClick(View v) { LocationViewers.mapView.getController().zoomOut(); } }); } /** * Must let Google know that a route will not be displayed */ @Override protected boolean isRouteDisplayed() { return false; } }
While pressing the zoom controls plus or minus, this listener will be called and the zoom functionality will be occurs. Then we are going to see two filesMapLocation.java and MapLocationOverlay.java, where in the MapLocationOverlay file we will be drawing the marker and the info window for each marker, you can get the code from the zip file below.
The main thing to run the application is adding the internet permission and google library in the AndroidManifest.xml file
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.icons.draw"
android:versionCode="1"
android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".DrawIcons"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<uses-library android:name="com.google.android.maps" />
</application>
<uses-permission android:name="android.permission.INTERNET" />
<uses-sdk android:minSdkVersion="4" />
</manifest>
Thanks
akm from cdacians(www.cdacians.com)
Cdacians, Great post, could you please send me the source code for the same.
ReplyDeleteHey please see the post i have all ready mention the source code :)
ReplyDeleteCdacians, please upload complete source code to download because there are few things we are missing, after complete source code i am dame sure this example will be the most useful for the developers....
ReplyDelete